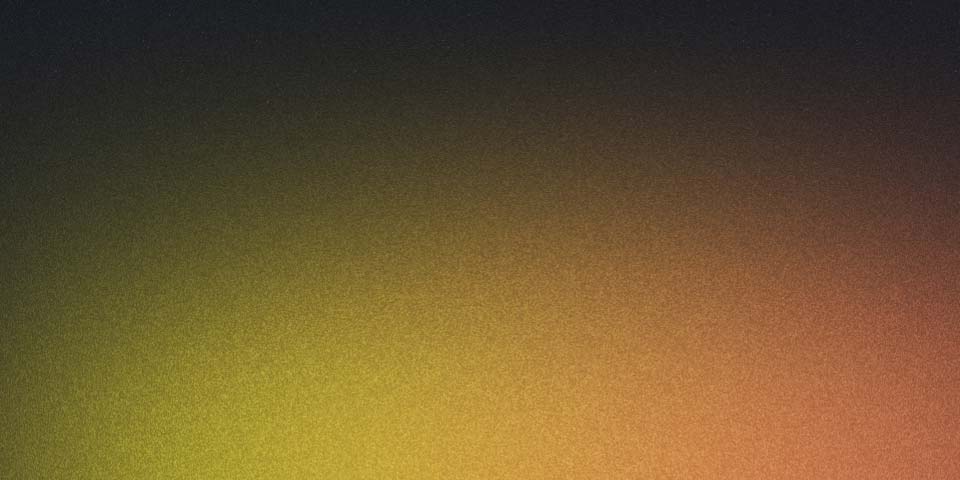
Mastering Functions in Rust
Functions are the building blocks of any programming language, and Rust is no exception. You could write hugely complex programs without ever leaving the main function, but that would be utter maddness and you would be compelled to wear a straightjacket.
In this tutorial, we’re going to take a deep dive into functions in Rust, covering everything from defining functions to closures and everything in between.
Defining Functions
Defining functions in Rust is a piece of cake. You can define a function using the fn
keyword followed by the function name, a list of parameters, and the return type. Here’s an example:
fn add(a: i32, b: i32) -> i32 {
a + b
}
Let’s break this down a little and focus on the different parts of the function definition:
fn
: This is the keyword used to define a function in Rust.add
: This is the name of the function.(a: i32, b: i32)
: These are the parameters that the function takes. In this case, the functionadd
takes two parameters,a
andb
, both of typei32
.-> i32
: This is the return type of the function. In this case, the functionadd
returns a value of typei32
.
The body of the function is the expression a + b
, which adds the two parameters together and returns the result.
Calling Functions
Once you’ve defined a function, you can call it from other parts of your code. Here’s an example of calling the add
function we defined earlier:
fn main() {
let result = add(5, 10);
println!("The result is: {}", result);
}
In this example, we call the add
function with the arguments 5
and 10
, and store the result in a variable called result
. We then use println!
to print the result to the console.