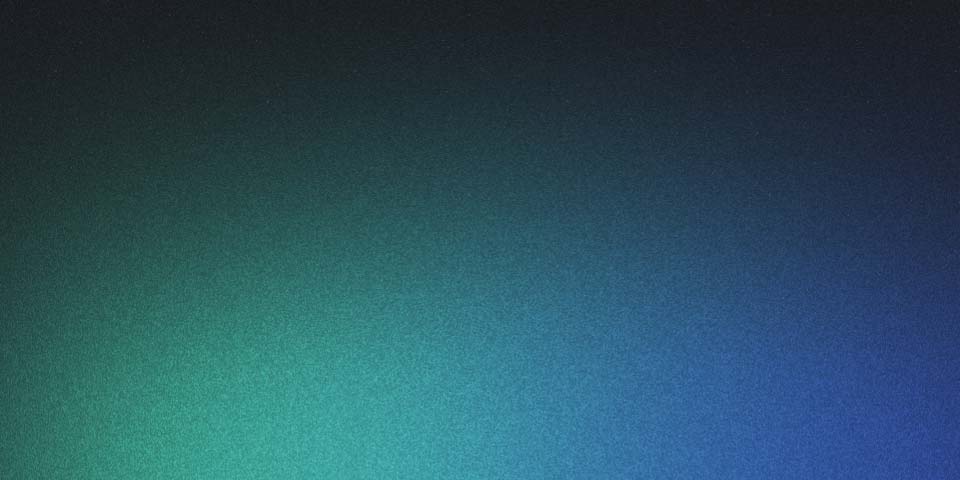
Getting Started with Modules in Rust
There comes a point in every Rust application’s life where it starts to grow out of control. The main.rs
file becomes a behemoth of a file, and you start to lose track of where everything is. You start to wonder if there’s a better way to organize your code.
Well, fear not! Rust has a solution for you: modules!
What Are Modules?
Modules are a way to organize your code in Rust. They allow you to group related code together, making it easier to manage and understand. Modules can contain functions, structs, enums, and other modules, allowing you to create a hierarchy of code that mirrors the structure of your application.
As your application grows, you should start to think about how you can break it down into smaller, more manageable pieces. You’ll also need to think about how you ensure that each of these pieces is functionally decoupled from the others and how they can be tested in isolation.
Creating a Module In A Rust File
Option 1 when breaking your code into modules is to create the module in the same file as your main.rs
or lib.rs
file. This is the simplest way to create a module in Rust.
Here’s an example of how you can create a module in the same file as your main.rs
:
mod my_module {
pub fn hello() {
println!("Hello from my_module!");
}
}
fn main() {
my_module::hello();
}
Note: we’ve explicitly made the
hello
function public by using thepub
keyword. This means that it can be accessed from outside the module. Functions are private by default in modules in Rust.
Creating a Module In A Separate File
Option 2 - when breaking your code into modules is to create the module in a separate file. This is useful when your module starts to grow and you want to keep your codebase clean and organized.
Here’s an example of how you can create a module in a separate file:
// src/hotel.rs
pub fn welcome_guest() {
println!("Hello from the hotel!");
}
In the main.rs
file, you can then use the module like this:
mod hotel;
fn main() {
hotel::welcome_guest();
}
Creating Modules in Sub Directories
Let’s imagine you were building a hotel management system and you wanted to create a module for each part of the hotel. You could create a directory structure like this:
src/
main.rs
hotel/
mod.rs
front_desk.rs
housekeeping.rs
kitchen.rs
In the hotel/mod.rs
file, you would define the modules like this:
pub mod front_desk;
pub mod housekeeping;
pub mod kitchen;
And in the front_desk.rs
file, you would define the front_desk
module like this:
pub fn check_in() {
println!("Checking in a guest!");
}
You could then use the modules in your main.rs
file like this:
mod hotel;
fn main() {
hotel::front_desk::check_in();
}