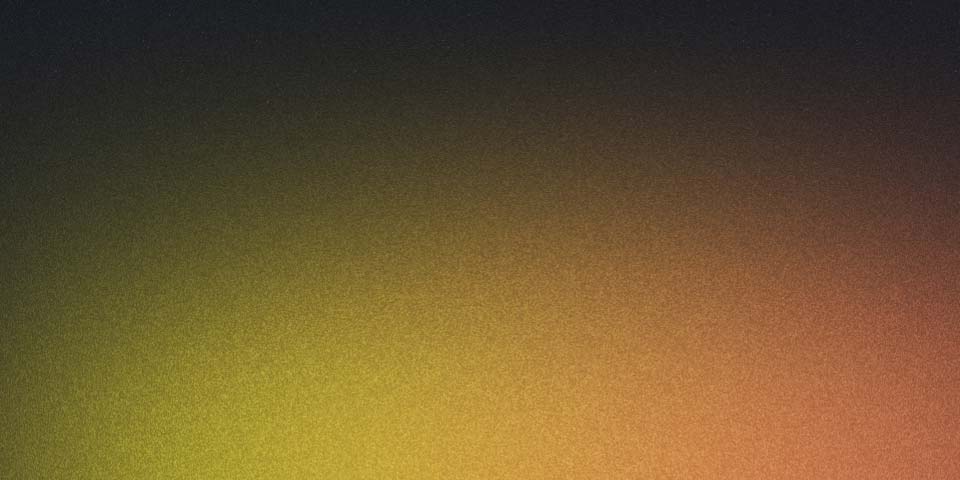
Building a Simple Server With Rust and Axum
I enjoy building things with a language in order to learn it, and I’m sure you do too. So, let’s build a simple server with Rust and Axum.
What is Axum?
Axum is a web framework built on top of hyper and tower. It is designed to be both easy to use and performant.
Setting Up Your Project
First, you’ll need to create a new Rust project. You can do this by running the following command:
cargo new simple_server
Next, you’ll need to add the axum
and tokio
dependencies to your Cargo.toml
file:
[dependencies]
axum = "0.7.5"
tokio = { version = "1", features = ["full"] }
Creating Your Server
Now that you have your project set up, you can start building your server. Open up a file called main.rs
in the src
directory of your project and add the following code:
use axum::{routing::get, Router};
#[tokio::main]
async fn main() {
let app = Router::new().route("/", get(|| async { "Hello, World!" }));
let listener = tokio::net::TcpListener::bind("0.0.0.0:4000").await.unwrap();
axum::serve(listener, app).await.unwrap();
}
Awesome, you’ve just created a simple server that listens on port 4000
and responds with Hello, World!
when you navigate to http://localhost:4000
.
Let’s break it down Now
We start by importing the necessary modules from the axum
crate.
use axum::{routing::get, Router};
We define a main
function that is marked as async
using the #[tokio::main]
attribute.
#[tokio::main]
async fn main() {
We create a new Router
using the Router::new()
function and define a single route that responds with Hello, World!
when the root path is accessed.
let app = Router::new().route("/", get(|| async { "Hello, World!" }));
We bind the server to localhost:4000
using tokio::net::TcpListener::bind("0.0.0.0:4000").await.unwrap()
.
let listener = tokio::net::TcpListener::bind("0.0.0.0:4000").await.unwrap();
Finally, we serve the app using axum::serve(listener, app).await.unwrap()
.
axum::serve(listener, app).await.unwrap();
Running Your Server
To run your server, you can use the following command:
cargo run
You should see the following output:
Compiling simple_server v0.1.0 (/Users/elliotforbes/simple_server)
Finished dev [unoptimized + debuginfo] target(s) in 0.23s
Running `target/debug/simple_server`
Now, navigate to http://localhost:4000
in your browser, and you should see Hello, World!
displayed on the page.